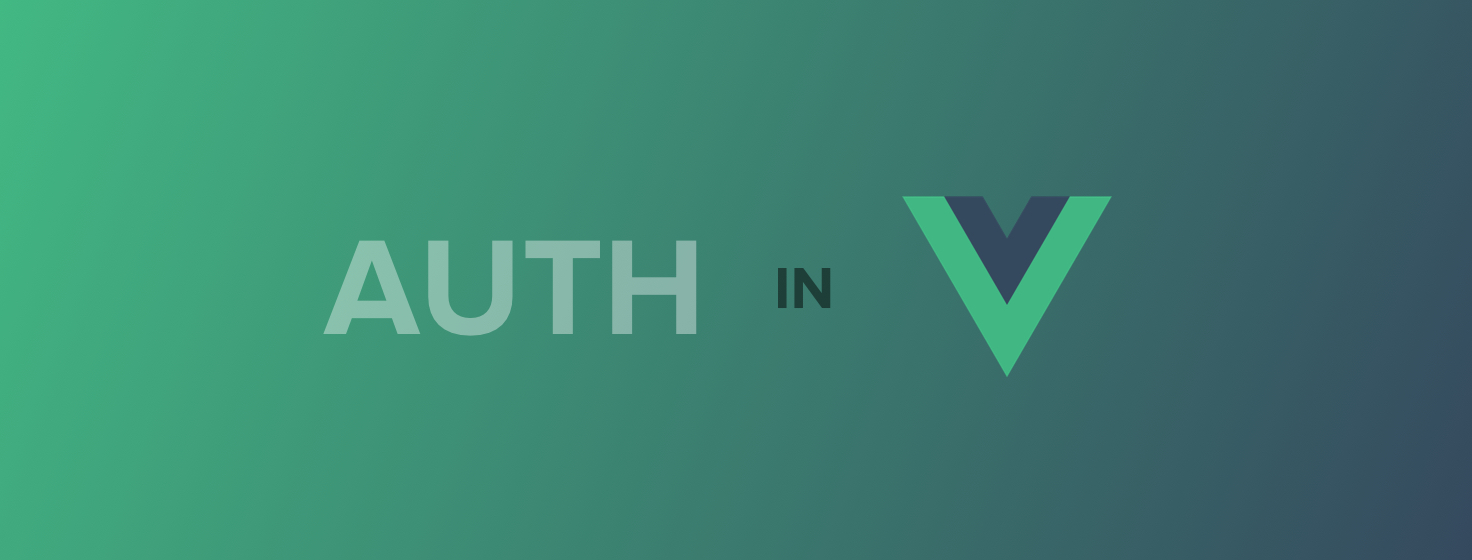
Vue: Authentication Best Practices
Learn how to easily implement authentication in your Vue.js application. You will learn how to handle unauthenticated API calls, have auto authentication, have restricted routes access and more.
Learn how to easily implement authentication in your Vue.js application. You will learn how to handle unauthenticated API calls, have auto authentication, have restricted routes access and more.
In authenticated frontend apps we can change what a user can see depending on their role e.g. guest, admin etc. In this article, we implement a simple but scalable permission system into a Vue.js app using the CASL library.
Atomic Design creates multiples stacks of components, with different hierarchies of complexity and dependence
Frontend developers utilize a variety of tools. Discussion of these tools and arguing which one is the best will easily grow in a holy war, as every developer has his own preferences in terms of methods of working and using programming languages. That is why the wisest decision one can make is to
Deciding on a JavaScript framework for your web application can be overwhelming. Angular and React are very popular these days, and there is an upstart which has been getting a lot of traction lately: VueJS
This is the official style guide for Vue-specific code. If you use Vue in a project, it’s a great reference to avoid errors, bikeshedding, and anti-patterns.
Chad shows how to conditionally apply a CSS class at runtime, binding to a JS object by defining a class and creating class bindings in your template.
Anyone who has used the flux architecture in their apps, be it Vuex, Redux or another, knows a lot of boilerplate comes with it. Let’s see…
One of the cool things about native JavaScript modules for Vue.js users is that they allow you to organize your components into their own files without any kind of build step required. In this article, I'm going to show you how to write a single-file JavaScript component without any Babel or Webpack!
Do you have components in your Vue.js app that share similar options, or even template markup? In this article I'll show you a design pattern for extending Vue components that'll help keep your code DRY.
It's a common practice for a Vue app to use the DOM as its template. In this article, I'll explain the issues and offer some alternatives.
All you need to know about Vue.js Filters. Can be useful in a lot of different situations like keeping your API responses clean and handling the formatting of your data on your frontend.
This course will show how to take full advantage of TypeScript static typing on Vue apps by using class based components, typing directives and using decorators.
Is jQuery really easier to learn than Vue.js? In this article we show you why Vue.js might be a better alternative for beginners.
Not all browsers have client-side validation built-in and all of them behave differently. Let’s look at how to build our own form validation using Vue.js so it behaves the same in all modern browsers.