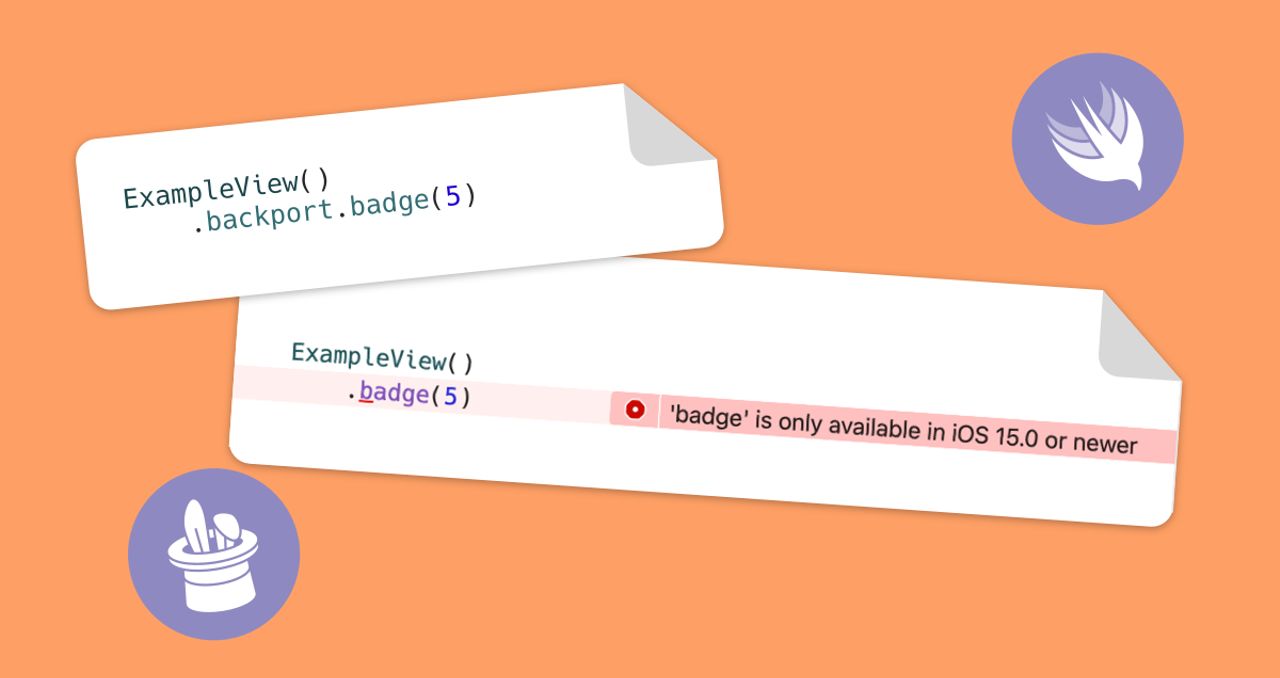
Checking for availability of iOS 15 in a SwiftUI view body
How to check for the availability of an iOS version in a SwiftUI View body to make use of new View modifiers that have been added in recent iOS releases.
How to check for the availability of an iOS version in a SwiftUI View body to make use of new View modifiers that have been added in recent iOS releases.
Learn from a Google Developer Expert: Core Data is a beautifully designed object mapping and persistence framework -- big kudos to the Apple engineers.
This tutorial zooms in on an important topic for anyone working with Core Data, faulting. Faulting is a concept that often confuses developer new to Core Data. Before I explain what faulting is, I want to show it to you.
How to build a Swift Package Plugin to automatically generate unit tests from input Swift files.
Combine's filter and compactMap operators share a few similarities and it is possible to use them interchangeably in some scenarios. That said, there are a number of key differences we discuss in today's episode of Combine Essentials.
Use control flow statements inside a WidgetBundle to return a different set of widgets base on a if-statement condition.
The @ViewBuilder attribute allows you to create compact code and improve readability. SwiftUI forces you already to use the result builder.
In this tutorial I will try to present you how to use clean, testable and maintainable architecture when using SwiftUI. We start with diagram depicting this architecture and then we dive deep into…
Add RawRepresentable conformance to a custom type that represents a setting or a view state in SwiftUI to be able to save it in AppStorage or SceneStorage.
Since Apple introduced SF Symbols in 2019, many product designers for iOS, including myself, have been asking themselves when it will
Everything you need to know about the new application life cycle in SwiftUI 2
There seems to almost be a consensus in the community that singletons are
Dependency injection is an essential tool when it comes to making code more testable. This week, let's take a look at a dependency injection technique that lets us enable testability without forcing us to write massive initializers or complicated dependency management code.
How the MainActor attribute eliminates the need for us to manually dispatch UI updates on the main queue when using Swift 5.5’s new concurrency system.
Explaining the Dependency Injection pattern, by contrasting it with Service Locator. The choice between them is less important than the principle of separating configuration from use.